Disclaimer: This article is written based on Windows 10 Tech Preview – Build 10122. Things might change completely in the future.
Some of the Windows 10 apps have a back button at the top of the app bar. Here’s an example in the Settings Control Panel:
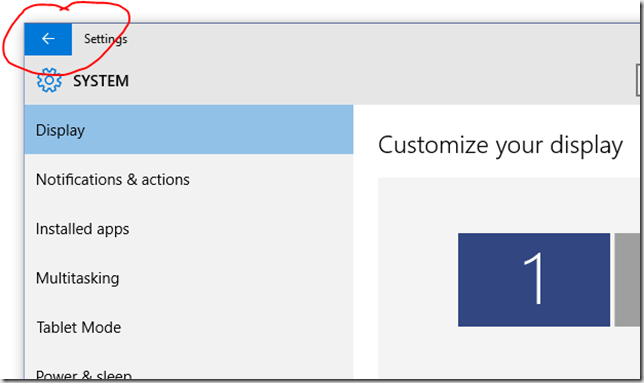
We can add this to our own apps but using the SystemNavigationManager’s AppViewBackButtonVisibility.
Here’s how that will look like:
SystemNavigationManager.GetForCurrentView().AppViewBackButtonVisibility = AppViewBackButtonVisibility.Visible;
When you run this code you’ll now also see the exact same back button in your Windows UWP Desktop app! No need to waste precious screen real-estate when there’s room on the top bar.
Of course for Windows Phone this isn’t really needed (although nothing seems to happen if you call this API), call it anyway, just in case you're running on a device that can show the backbutton - it seems like it just doesn't show on devices with hardware:
//Show UI back button - do it on each page navigation
if (Frame.CanGoBack)
SystemNavigationManager.GetForCurrentView().AppViewBackButtonVisibility = AppViewBackButtonVisibility.Visible;
else
SystemNavigationManager.GetForCurrentView().AppViewBackButtonVisibility = AppViewBackButtonVisibility.Collapsed;
//Hook up back buttons - Do this just once - ie on app launched
SystemNavigationManager.GetForCurrentView().BackRequested += (s, e) =>
{
if (Frame.CanGoBack)
Frame.GoBack();
};
if (Windows.Foundation.Metadata.ApiInformation.IsTypePresent("Windows.Phone.UI.Input.HardwareButtons"))
{
//Also use hardware back button
Windows.Phone.UI.Input.HardwareButtons.BackPressed += (s, e) =>
{
if (Frame.CanGoBack)
{
e.Handled = true;
Frame.GoBack();
}
};
}
Now you get back button support in both desktop and phone apps when you can navigate back!
Unfortunately the BackRequested and BackPressed event arguments are different, so you can’t reuse the same event handler for both. That’s quite a shame – I hope Microsoft will be cleaning that up soon.